springboot中异常处理的几种方式:
1).使用默认springBoot中默认的异常处理:BasicErrorController.class
2).自定义异常处理类
3).使用@ExceptionHandler注解做全局异常处理
1.自带的异常处理分为2类一种是返回页面,一种是返回json格式如下:
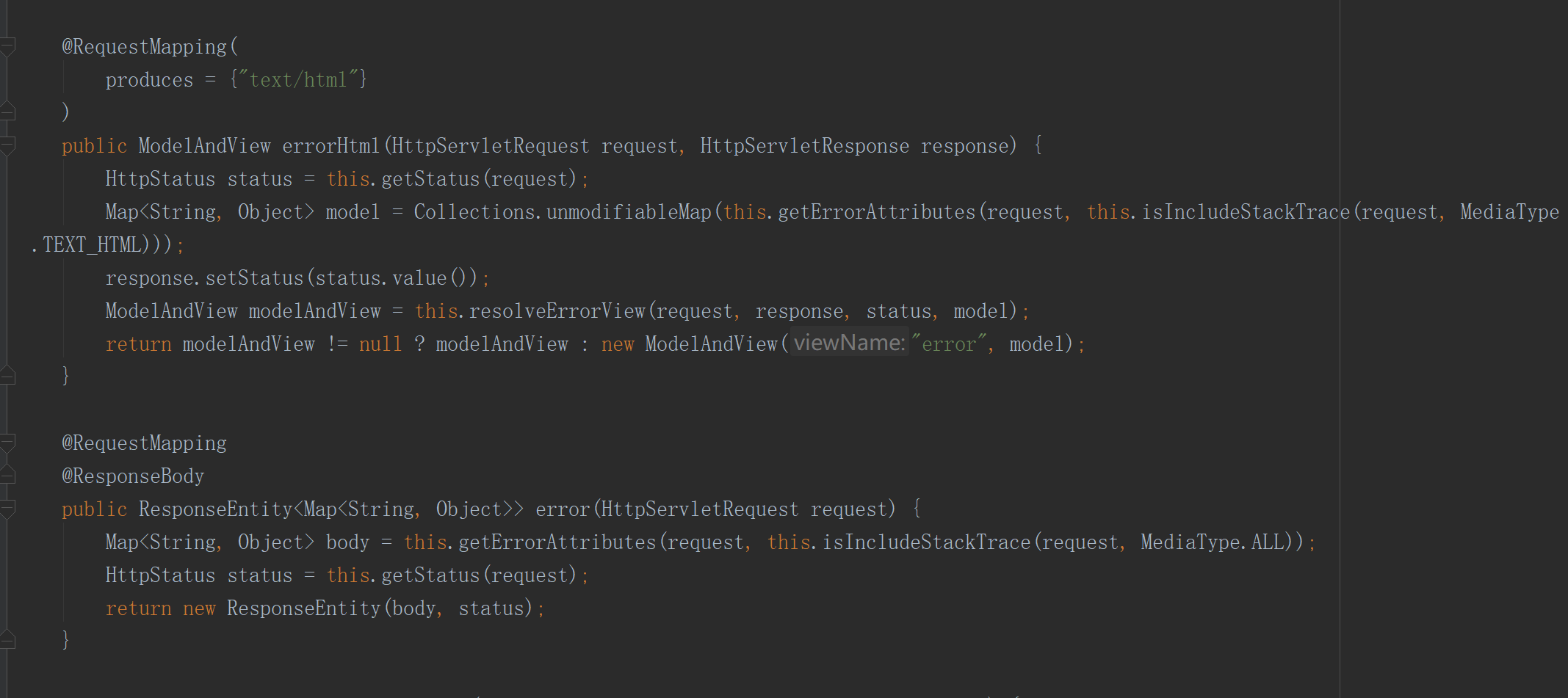
我们可以使用自定义的异常处理类和错误配置页面来覆盖的默认的错误提示页面如下:
1 |
|
2.使用@ExceptionHandler注解做全局异常处理
1 |
|
3.若只返回json数据我们也可以继承BasicErrorContorller类,重写error方法编写自己想要的数据如下:
1 |
|