什么是Web Services:
- Web Services 是应用程序组件
- Web Services 使用开放协议进行通信
- Web Services 是独立的(self-contained)并可自我描述
- Web Services 可通过使用UDDI来发现
- Web Services 可被其他应用程序使用
- XML 是 Web Services 的基础
它如何工作?
基础的 Web Services 平台是 XML + HTTP。
HTTP 协议是最常用的因特网协议。
XML 提供了一种可用于不同的平台和编程语言之间的语言。
Web services 平台的元素(三要素):
- SOAP (简易对象访问协议)
- UDDI (通用描述、发现及整合)
- WSDL (Web services 描述语言)
一:搭建Web Service服务
使用的技术:Springboot1.5.17.RELEASE版本,无需创建Web项目简单的java项目就行。
在确保springboot项目正常运行的情况下需要额外导入如下jar:
1 |
|
下面正式开始WebService的流程搭建:
1.创建一个接口类:
1 |
|
2.创建接口类的实现类:
1 |
|
注:endpointInterface为接口类,targetNamespace和wsdl文档中的targetNamespace一致(否则在接口调用的时候可能会报错,至于这个地址怎么写在启动服务后可以查看是否一致不一致则修改查看的方法后面会提到)。
3.创建webService实体配置文件
1 |
|
5.启动App程序
1 |
|
6.启动成功后的步骤
1.在页面打开:http://localhost:8080/service/user?wsdl 会出现如视图:
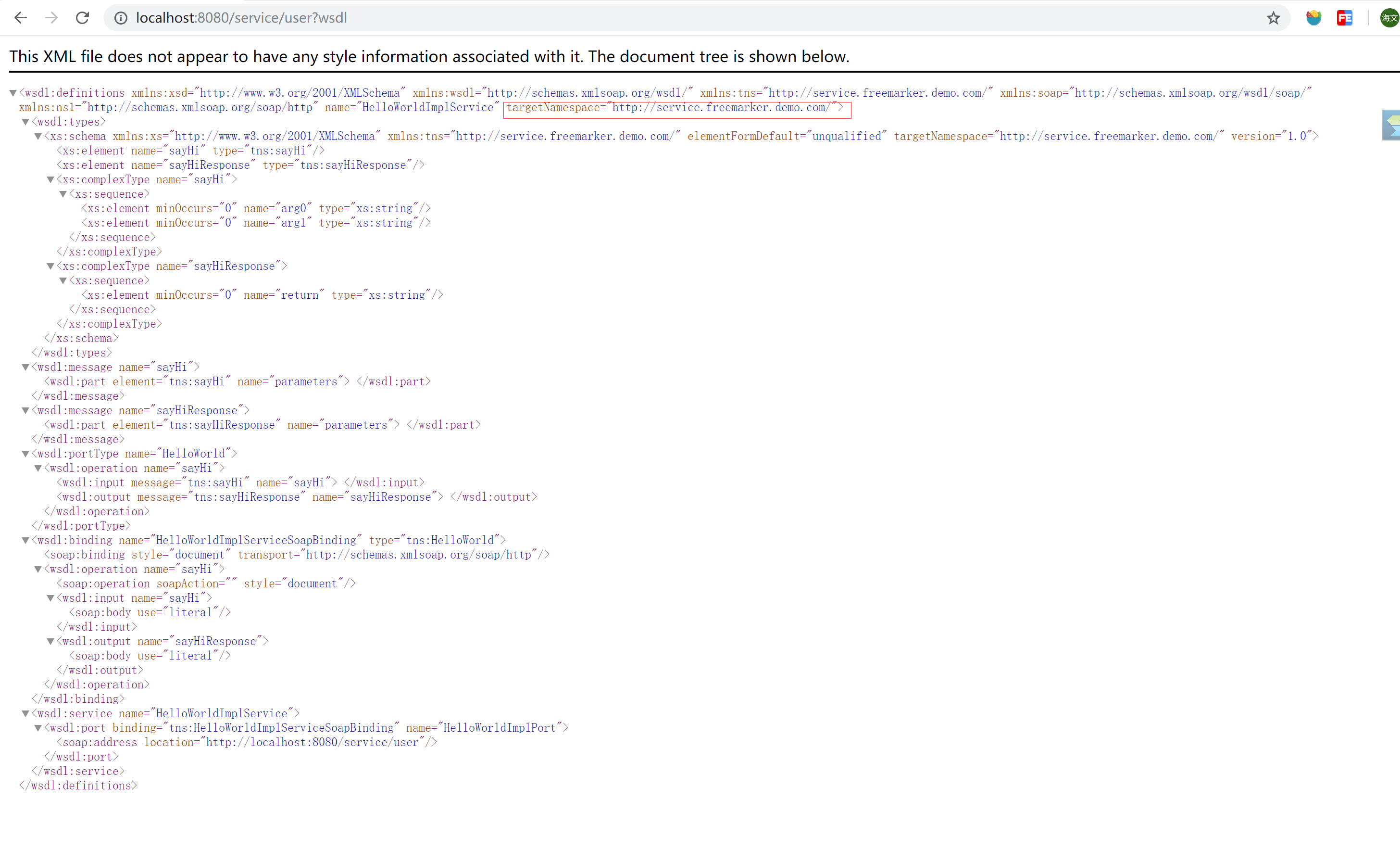
注:其中targetNamespace的值应和上面实现类的targetNamespace值一致
至此简单WebService服务到此创建完成。
二:调用WebService服务上的接口
调用的方法有很多种这里说的是org.apache.axis.client中的Call方法
1.引入jar包:
1 |
|
2.创建一个调用类
1 |
|